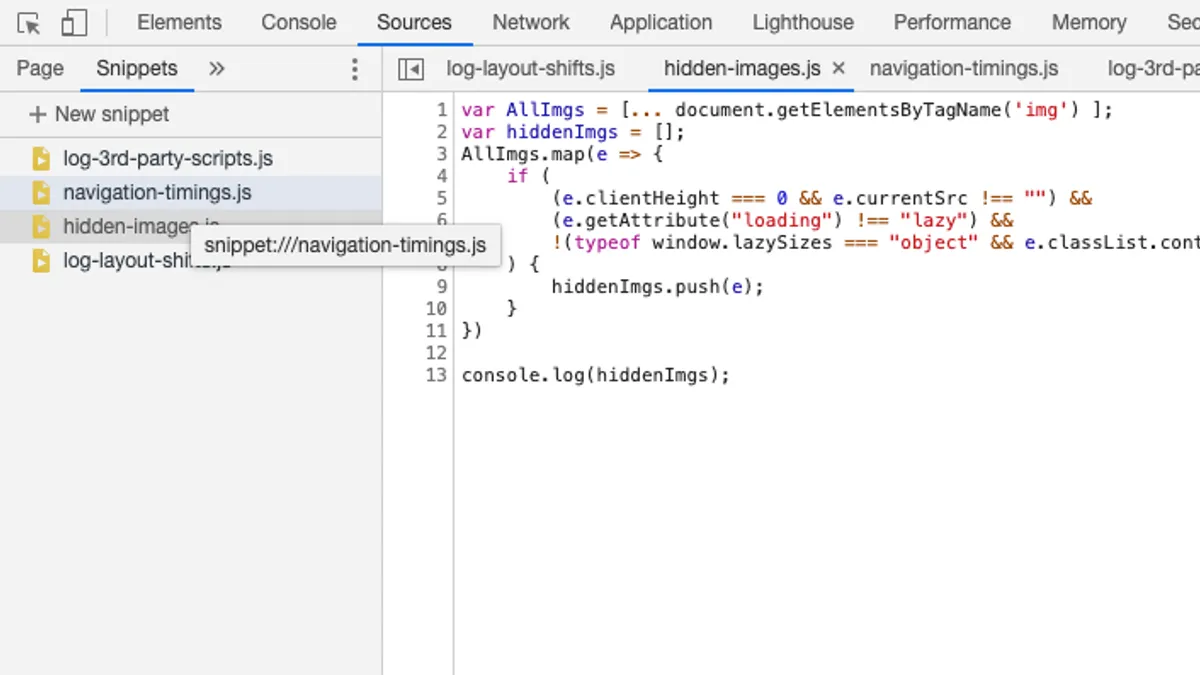
DevTools Snippets
Run small snippets of JavaScript code quickly within Chrome DevTools
Chrome DevTools is a remarkable tool with no doubt that offers a collection of features and tools within its interface that I have strong reason to believe that most of them are not very well known. But they are absolute gems 💎 !
One such amazing feature is the Snippets
section within the Sources
tab.
DevTools snippets allow you to execute small (or as big as you might like) snippets of JavaScript code, just like you would write any code within the Console
tab.
Using DevTools Snippets#
Opening Snippets#
Firstly to locate the Snippets
section within Chrome DevTools, you will have to navigate to the Sources
tab.
If you cannot find the tab in the DevTools interface, just type ⌘ + ⇧ + P to bring up the command palette and type/select "sources".
Creating a new snippet#
To create a new snippet, simply click on the "+ New snippet" button and give it a name.
Then write the code on the right hand side panel that you would like to execute each time you are running that snippet.
Warning: Don't forget to hit save by pressing ⌘ + S !
Execute a snippet#
Now there are a few ways to execute a snippet (four to be precise) and let's look at all of them below, then you can decide which is more convenient and faster.
The most obvious way is to run it straightaway within the Snippets
section where we created it. At the bottom of the screen press the play button ▶️ to execute the script.
As the button suggests you can also run it by pressing ⌘ + enter while in the Snippets
section.
An easier and more convenient way to execute a snippet is by pressing ⌘ + P and typing "!". Following the exclamation mark, you will see some autocomplete values. Type to select the name of your snippet and hit enter ! Boom!
Note: There is no need to be inside theSnippets
section to execute it using the!<SNIPPET NAME>
command.
Now the last way is my favourite (because it's the laziest 😊) but i requires following a few steps before running a snippet. It is by actually creating a bookmarklet like this:
- Copy to clipboard the JavaScript you want to run as a snippet
- Create a new bookmark from the browser options menu (on Chrome press ⌘ + D and press "More...")
- In the URL field, type
javascript:
and paste the code you copied on step 1 - Finally hit "Save"
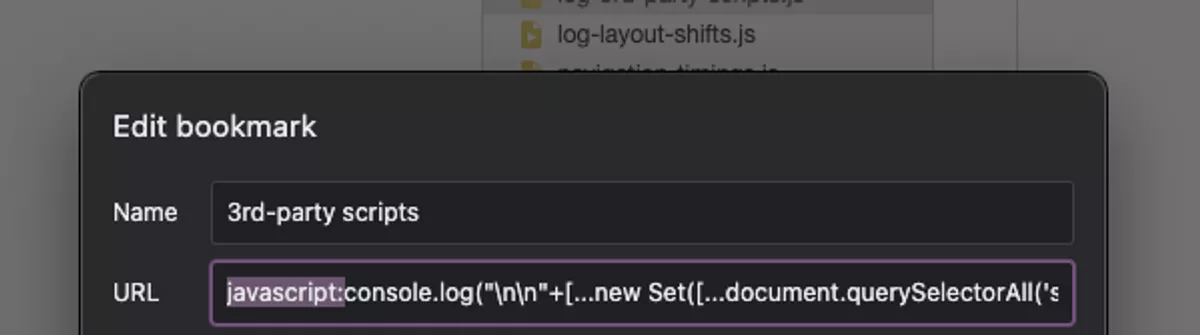
Voila! You can now simply hit the bookmarklet from your bookmarks bar and run a snippet instantly!
Handy snippets#
Lastly, let me share some of my most handy snippets in this page, that I think you will find useful especially if you are a site speed geek like me 🤓
Snippet
Log hidden images#
var AllImgs = [... document.getElementsByTagName('img') ];
var hiddenImgs = [];
AllImgs.map(e => {
if (
(e.clientHeight === 0 && e.currentSrc !== "") &&
(e.getAttribute("loading") !== "lazy") &&
!(typeof window.lazySizes === "object" && e.classList.contains("lazyload"))
) {
hiddenImgs.push(e);
}
})
console.log(hiddenImgs);
Snippet
Log Layout Shifts#
new PerformanceObserver((list) => list.getEntries().map(console.log)).observe({type: 'layout-shift', buffered: true})
Snippet
Log navigation timings#
const entries = ["largest-contentful-paint", "navigation", "paint"];
entries.map(entry => {
const po = new PerformanceObserver((list) => {
for (const entry of list.getEntries()) {
console.log(entry.toJSON());
}
});
po.observe({type: entry});
})
Snippet
Log 3rd party scripts#
"\n\n"+[...new Set([...$$('script')].filter(e => !e.src.includes(document.location.hostname) && e.src !== "" && !e.src.includes('cloudfront') ).map( e => new URL(e.src).host))].join(' ')+"\n\n";
Snippet
Clear page#
Clear current document before recording a Performance timeline on reload in Chrome DevTools.
document.documentElement.innerHTML = '';
for (const obj of [document, window]) {
for (const event of Object.values(getEventListeners(obj))) {
for (const {type, listener, useCapture} of event) {
obj.removeEventListener(type, listener, useCapture)
}
}
}
Snippet
Log Cache storage#
let cacheNames = await caches.keys()
let cachesStorage = {}
let promises = cacheNames.map(async cacheName => {
const cache = await caches.open(cacheName)
const cachedResources = await cache.keys()
let storage = 0
for (const request of cachedResources) {
const response = await cache.match(request)
const blob = await response.blob()
storage += blob.size
}
return cachesStorage[cacheName] = storage
})
await Promise.all(promises)
console.table(cachesStorage)
Snippet
List event listeners#
console.table((function listAllEventListeners() {
const allElements = Array.prototype.slice.call(document.querySelectorAll('*'));
allElements.push(document); // we also want document events
const types = [];
for (let ev in window) {
if (/^on/.test(ev)) types[types.length] = ev;
}
let elements = [];
for (let i = 0; i < allElements.length; i++) {
const currentElement = allElements[i];
for (let j = 0; j < types.length; j++) {
if (typeof currentElement[types[j]] === 'function') {
elements.push({
"node": currentElement,
"type": types[j],
"func": currentElement[types[j]].toString(),
});
}
}
}
return elements.sort(function(a,b) {
return a.type.localeCompare(b.type);
});
})());
I once wrote a massive snippet that I could run from within DevTools so that I could style and alter an HTML document before downloading it again and using it for the pwa.js.org website.