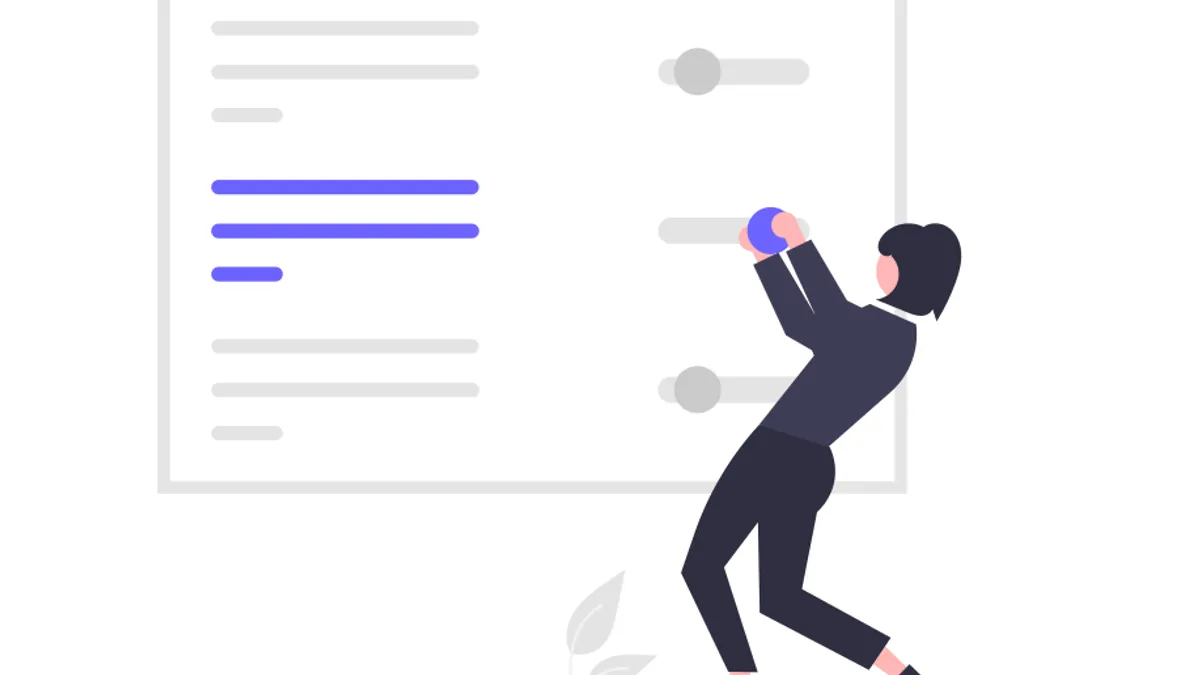
Illustration by unDraw
Environment variables and Babel
How to configure environment variables inside of babel.config.js
.
In this short guide we will look at a simple way of configuring our environment variables and load them for use inside of our front-end code, while using Babel.
Lastly we will look at a quick way to access those variables inside of the babel.config.js
file itself.
Configure env variables with Babel#
For this purpose we can use babel-plugin-inline-dotenv to load the corresponding env file based on what environment we are in, i.e. production vs development:
- Install the plugin with
npm install babel-plugin-inline-dotenv
- Include the plugin and the path to the
.env
file in your Babel config file:
babel.config.js
module.exports = function() {
return {
presets: ['@babel/preset-react'],
env: {
production: {
plugins: [["inline-dotenv",{
path: '.env.production'
}]]
},
development: {
plugins: [["inline-dotenv",{
path: '.env.development'
}]]
}
}
};
};
After this you should be able to access your variables you declared inside of your .env
file(s) in your JavaScript front-end code.
For example, in your .env.production
or .env.development
files add variables like this:
.env.development
API_KEY='<YOUR_API_KEY>'
Later in your code you can access the above variable like this:
app.js
process.env.API_KEY
This way, depending on what environment you are in (production or development) you can access a specific API key set for that specific environment.
Note: The variables underprocess.env
can only be accessed directly (e.g. ✅process.env.API_KEY
) and cannot be accessed by de-structuring theprocess.env
object.
This will not work: ❌const { API_KEY } = process.env
Access env variables inside babel.config.js
#
To access our env variables within the babel.config.js
file, we can use dotenv
like this:
babel.config.js
require('dotenv').config({ path: './.env.development' })
console.log('API_KEY: ' + process.env.API_KEY)
module.exports = function() {
// ...
}