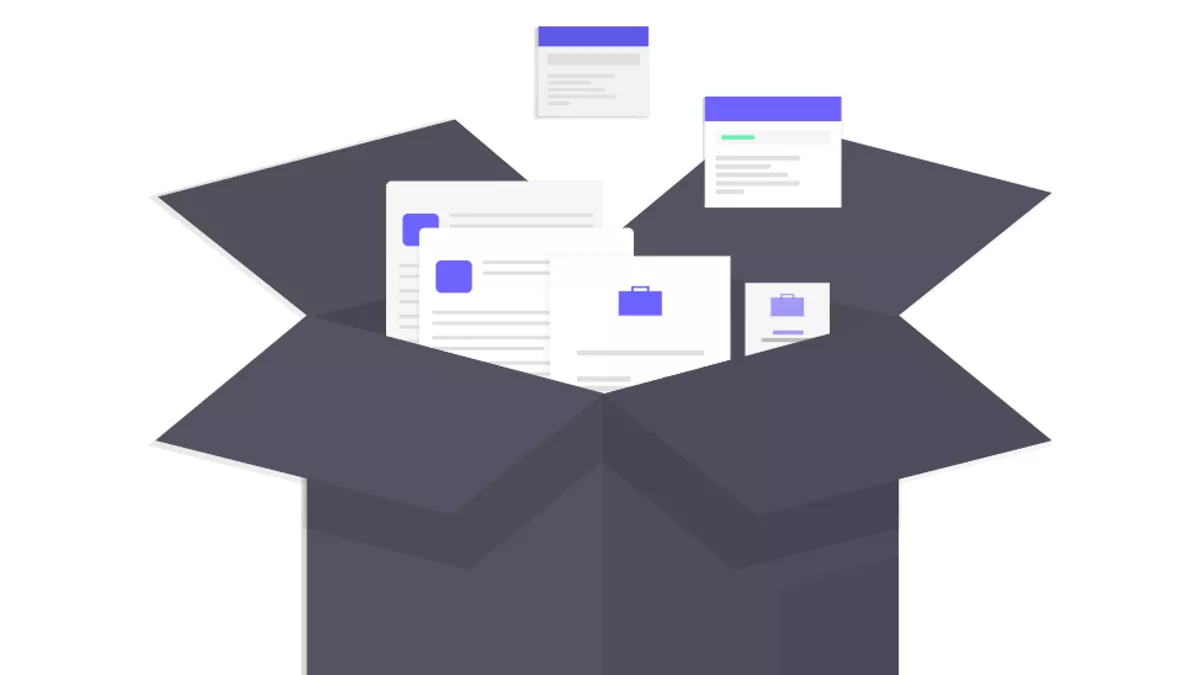
Illustration by unDraw
Workbox Strategies with examples and use-cases
Looking at how to use the Workbox library and its Strategies to improve any Progressive Web App.
When using service workers in a Progressive Web App and you’re already familiar with the APIs and the way everything works inside the service worker’s thread, you can definitely write all of the code yourself with vanilla JavaScript.
Although vanilla JS will give you more clarity, it’s still recommended that you use Workbox to avoid any mistakes and add simplicity to your code for better maintenance.
Workbox is a set of JavaScript libraries aiming to simplify implementation of advance caching using service workers and progressive web app development in general.
Workbox can simplify your service worker file, making it more readable and easy to update by using an error-free code beneath the hood.
Best practise: As I was starting out with Service Workers and PWAs I wanted to do everything vanilla so that I am sure I know what's going on. I definitely recommend this if you are a beginner to Service Workers - then you can move to something like Workbox to make your life easier 😀
In this short piece, I will be laying out a short and simple use-case for each of the Workbox strategies, aiming to enlighten you in case you got confused with these relatively new nuances or are just starting out with Workbox.
Workbox Strategies Explained#
Workbox strategies are used as ways of responding to a client’s HTTP requests. For example, let’s say the client asks for a resource with a URL /styles/main.css
. The strategy specified tells the service worker how to respond to this request. Should the service worker start looking for the /styles/main.css
resource in the cache first? Or from the network? Or both?
Examples and Use Cases#
To make sure you know which strategy best fits your case, let’s take a look at some use cases for all of the Workbox strategies available.
Cache first#
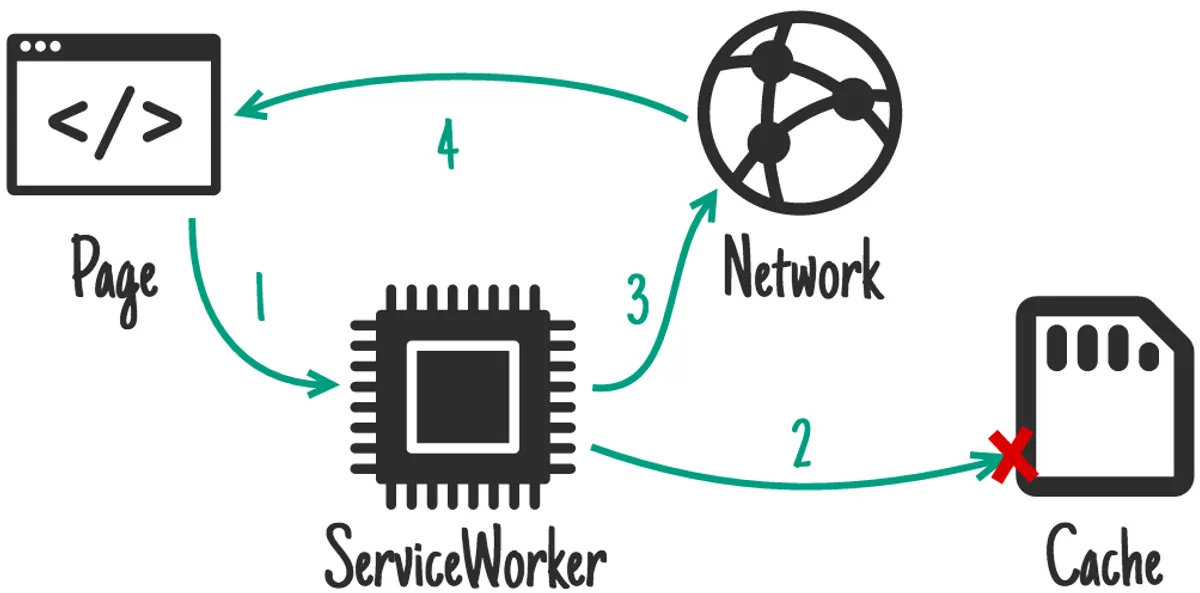
Try finding the resource in the cache first. If not there, try fetching it from the Network.
Use case:
- A resource whose version is not changing often, e.g., font files
Network first#
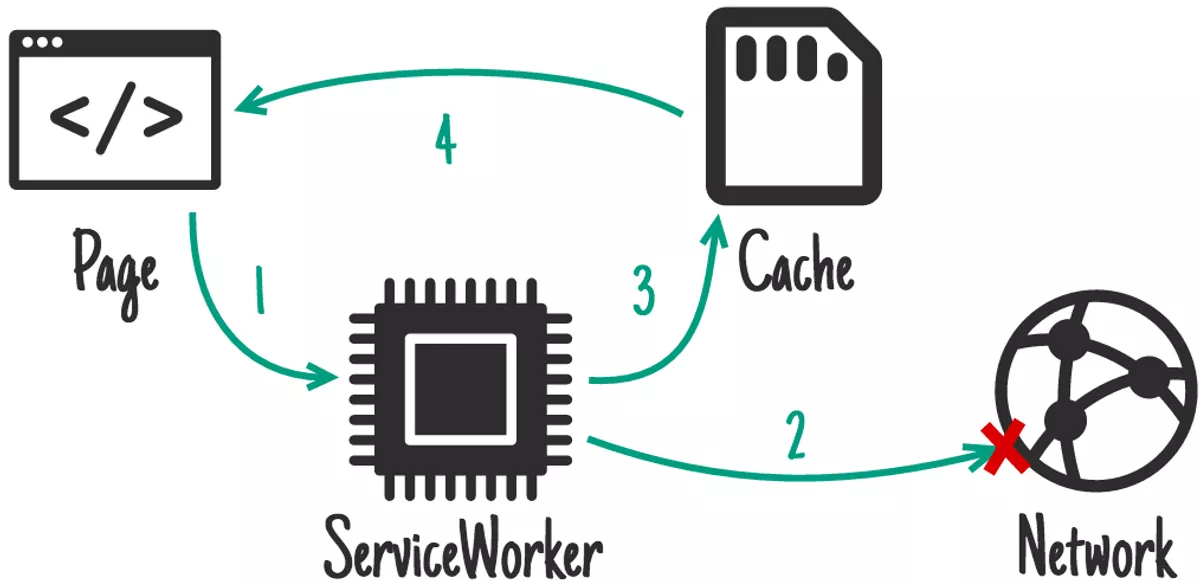
Try fetching it from the network first. If the resource isn’t found, try fetching it from the cache.
Use case:
- A resource whose latest version is important but still is needed offline, e.g., server-side-rendered HTML resources
Cache only#
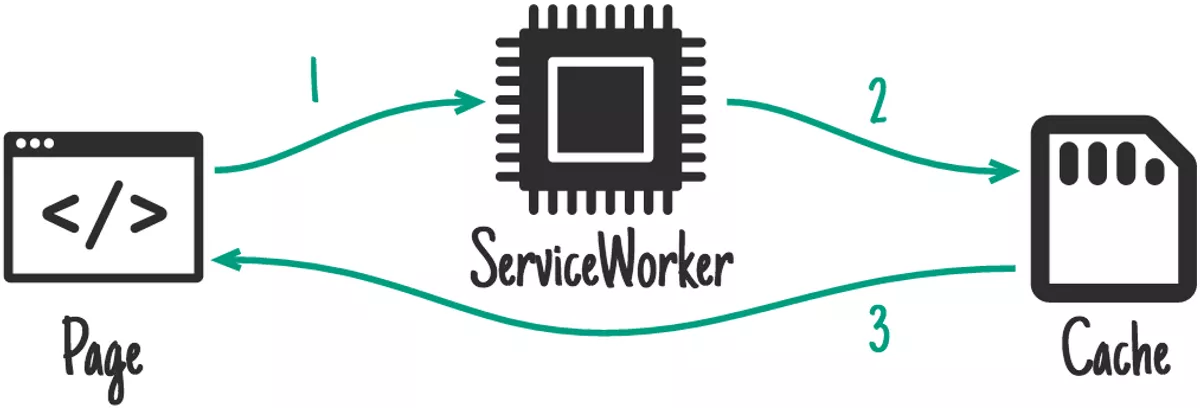
Try fetching the resource from the cache only. Return a failed request if not in the cache.
Use case:
- When you have already configured pre-caching for a file and want to use only that resource, e.g., a default offline page or a placeholder fallback image
Network only#
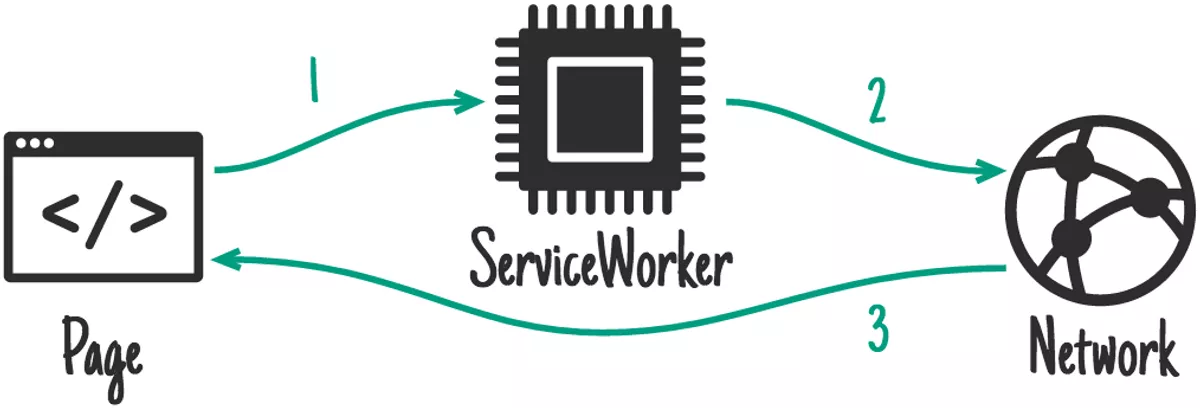
Try fetching the resource from the Network only. Return a failed request if URL was not found or during offline mode.
Use case:
- A page that only makes sense during an online live time, e.g., an active user page and its resources
Stale-while-revalidate#
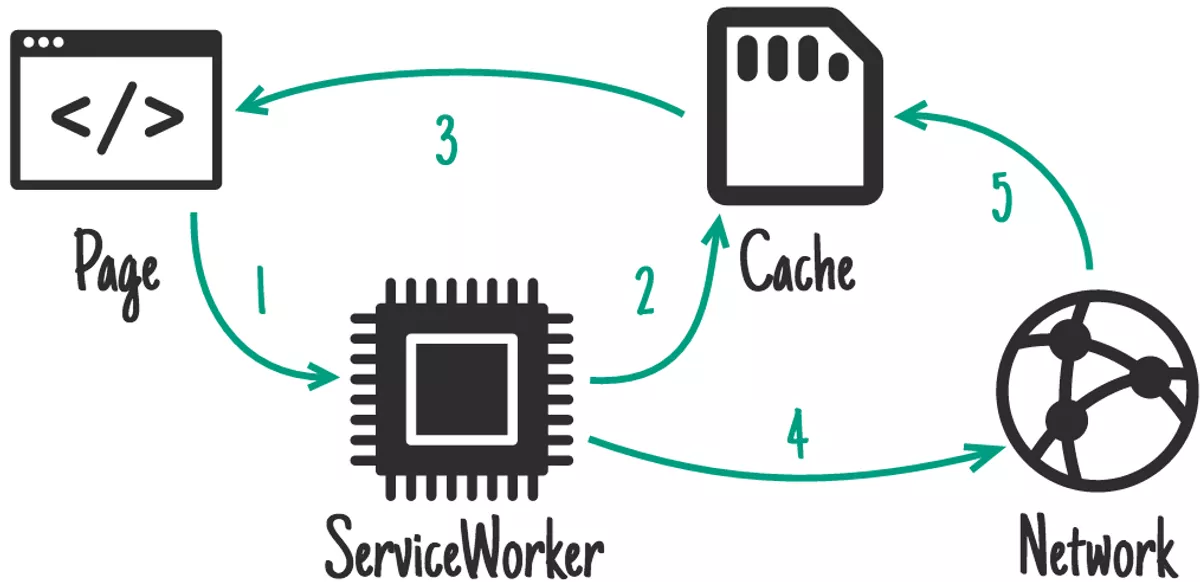
- Try fetching the resource from both the network and the cache.
- Respond from the cache if available and fall back to the network.
- Update the cache with the network response.
Use case:
- A resource whose freshness is not that important, but loading times have priority, e.g., CSS files for fast initial page rendering.
Applying Workbox Strategies#
Strategies are applied to specified HTTP routes. For this, Workbox provides a high-level interface through the registerRoute
function, which takes a RegExp
for matching multiple routes during run time.
This functionality allows route cache handling after adding a specific strategy based on all the routes’ URLs that match the RegExp
.
Stale-while-revalidate example#
sw.js
workbox.routing.registerRoute(
/\.(?:js|css|webp|png|svg)$/,
new workbox.strategies.StaleWhileRevalidate()
);
The above single-line code included in the service worker JavaScript file is stating that every request ending with any of the extensions js
, css
, webp
, png
, or svg
should be handled by the StaleWhileRevalidate
strategy.
When no strategy is specified, the default is used, which is cache first.
Network first example#
sw.js
workbox.routing.registerRoute(
/(\/|\.html)$/,
new workbox.strategies.NetworkFirst()
);
The above route registration is handy for server-side-rendered applications where it’s important to show the most up-to-date version of the website and database, but where you still want to show at least something to the user when there is no network.
If you would like to learn more about handling the Cache with JavaScript check out my other article 'Loading...'.