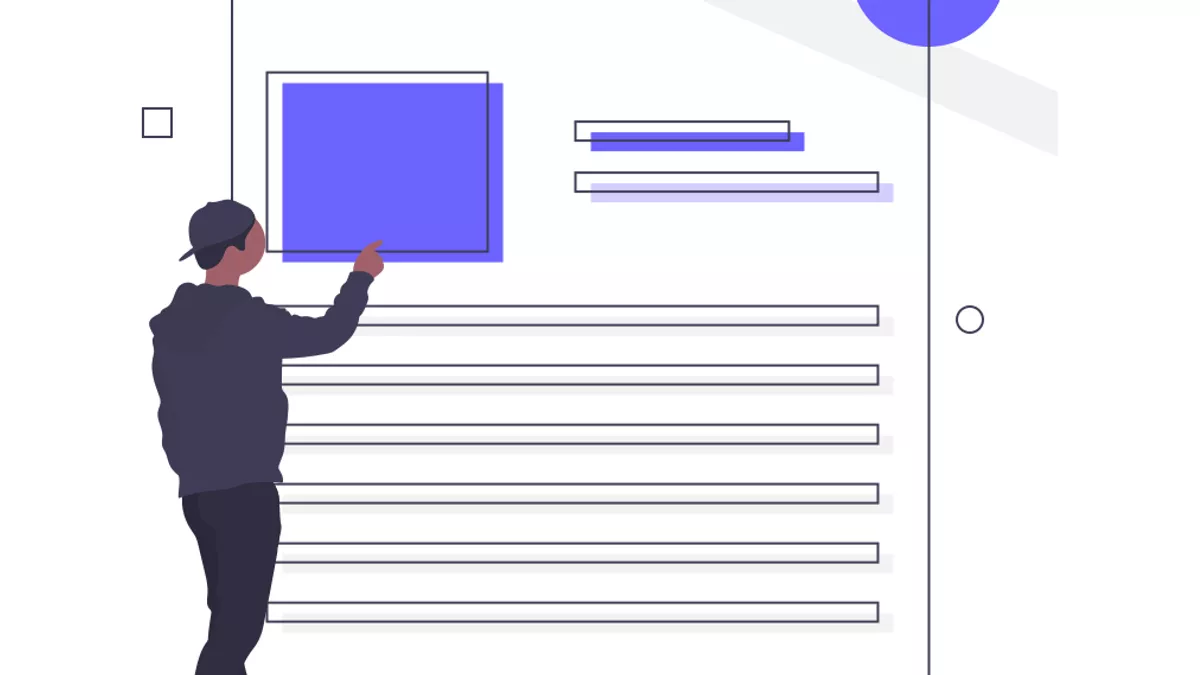
Illustration by unDraw
Fluid website scrolling with a tiny trick
Increase frames per second while users scroll a website with a tiny CSS trick.
In this article, I will be showing you a way you can improve your website’s scrolling performance - making it appear more fluid while a user scrolls down a page - and it might be one you have hopefully never heard of. The best part is that this optimization technique is rather simple to understand and implement, however it is only applicable on desktop I'm afraid.
Best practise: The following technique is useful because it minimises DOM reflow/layout and paint events, thus increasing the frame rate.
No hover effects while scrolling#
The goal here is to minimise browser reflow and paint events to increase the frame rate. As you might have guessed from the title, this is done by removing the hover
effect from all the elements in the DOM while a user is scrolling.
Let me elaborate in my next point why and where this is useful.
Let’s say you have this CSS rule on your page:
.color-me:hover {
background-color: blue;
}
This rule will be applied when the user’s cursor is hovering over it, which might happen while scrolling the page. It would then trigger a paint event in the browser as the element’s color has changed and so it will on every other element that has similar CSS rules.
The performance improvements and benefits are well explained in this brilliant demonstration by Ryan Seddon:
Implementation#
Scroll event listener#
const ENABLE_HOVER_DELAY = 500;
let timer;
window.addEventListener('scroll', function() {
const bodyClassList = document.body.classList;
// clear previous timeout function
clearTimeout(timer);
if (!bodyClassList.contains('disable-hover')) {
// add the disable-hover class to the body element
bodyClassList.add('disable-hover');
}
timer = setTimeout(function() {
// remove the disable-hover class after a timeout of 500 millis
bodyClassList.remove('disable-hover');
}, ENABLE_HOVER_DELAY);
}, false);
In an attempt to increase a website’s frame rate, we will attach a scroll
listener to the window
global object.
Every time there is a scroll, we clear the previous timeout and add the disable-hover
class to the <body>
element, so that it takes effect in the whole tree (hang in there for the next step).
Lastly, we set another timeout which will remove the disable-hover
class if the scroll
event is not called within 500 milliseconds (ENABLE_HOVER_DELAY
).
Tip: You can try out different values for the ENABLE_HOVER_DELAY
constant and see what fits your website best, but I found that 500 milliseconds is optimal.
CSS rule to disable all pointer events#
Lastly, we need to set this CSS rule for our listener to have an actual effect on our website while scrolling:
.disable-hover,
.disable-hover * {
pointer-events: none !important;
}
The above rule will ignore all pointer events on all the HTML elements.
Warning: In general, the user won’t be able to perform any mouse events (click
,hover
,drag
) while scrolling so make sure you understand the change in your website’s experience and that it doesn’t hurt the actual design or desired functionality of your website!
Conclusion#
It’s a quick and easy fix you can add to your website now, in an effort to optimise for speed by increasing the frame rate while scrolling. Go ahead. What are you waiting for?!
Hopefully you found this helpful. Cheers!